On any list, you can define a new column as a type of “Person or Group”. In event handlers or workflow, you may want to grab this value as use it as a real SPUser object, which will expose the name and email address, for example. Unfortunately if you just grab the value of the field, e.g.,
<br /> item["MyPersonField"]<br />
you’ll end up with a string that looks a bit like this:
15;#DOMAIN\Matt Thornton
Read more
A common problem in designing workflows is how to make the workflow “sleep” for a period whilst users do (or don’t do) something. A common scenario is where you wait for some user input, but if you don’t get any by a certain time to continue the workflow in a different stream. You achieve this with a delay activity where you specify a timeout in the form of a Timespan.
The delay activity was broken in SP2007 which was then fixed with a Hotfix. Surprisingly, this remains kind of broken in SP2010 as well. That is to say, the delay activity never wakes up.
Read more
Update: the below information is useful reference, but you may find it leads to undesirable results, where your workflowItem events fire repeatedly. Read the below, and then read this.
Working in Visual Studio 2010 and designing SharePoint 2010 state machine and sequential workflows, you may come across the following warning message when building and deploying your solution when using the onWorkflowItemChanged event:
Activity ‘onWorkflowItemChanged’ validation warning: The correlation token may be uninitialized
You will also probably notice that your workflow doesn’t respond or wake up to any onWorkflowItemChanged events. This is almost certainly due to the correlation token you’re using. The way to get this working correctly is:
Read more
This is one of those “d’oh” SharePoint moments. Created a new SharePoint feature and used Visual Studio to deploy it. Worked like a dream. Great, I think, ready for UAT. Package up the solution, install to the UAT environment and then attempt to activate the feature. Boom.
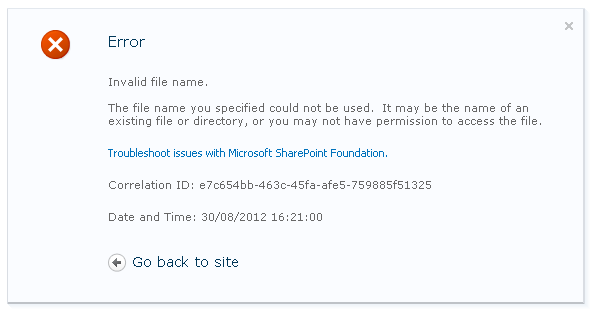
Weird. The feature was very simple so the error, as is the wont of SharePoint, was a bit unhelpful. Quick bit of Googling which didn’t help, before, thankfully, my brain engaged. I checked to see if what my feature was trying to do - create a site column and attach it to a couple of content types - had actually worked. It had.
Read more
This follows on from some of my other posts about deploying fields and content types programmatically. In this case, I was simply trying to add a choice field (SPFieldChoice) to a site, and then add it to some content types.
So I had something like:
var myCt = // snip - code to get my existing content type; // create the choice column var fieldChoices = new StringCollection() { "choice1", "choice2", "choice3" }; var docSourceField = web.Fields.Add("MyChoiceColumn", SPFieldType.Choice, false, false, fieldChoices); // get the column we just created SPFieldChoice docSourceChoiceField = (SPFieldChoice)web.Fields[DocumentSourceColumnName]; if (null == docSourceChoiceField) { throw new Exception("Error: Couldn't get the docSourceChoiceField."); } // add the new field to my content type myCt.Fields.Add(docSourceChoiceField); myCt.Update(true);
However, running this fell over with the error:
Read more
A while back I posted some info about using Event Receivers in SharePoint 2010 and a workaround to item values being null. Today, I needed to do something much more basic, and during an ItemUpdating event set the value of a column. I wrote the code that I knew would work - but, of course, it simply wouldn’t work. I did a spot of Googling about, and found the chaps at Tango Technology who had written a very simple solution to it. But - it wouldn’t work for me. I knew the event was firing - but no matter what I did, the new value just wouldn’t “take”.
Read more
I’m not a SharePoint administrator, but from time to time you have to get involved. I needed to migrate some data between farms but in order to do a backup / restore of e.g., Site Collections, version numbers have to be in range. Although the source server was reporting the version was OK, in Central Admin, it was saying that an upgrade was required. This is usually achieved by running the SharePoint 2010 Products Configuration Wizard.
Read more
If you encounter an issue, specifically in MySites, where you get an Access Denied error trying to edit the navigation, check whether you have the publishing feature enabled. I had this same issue when “Enterprise features” were re-enabled at the Central Admin, which for some reason enabled that on the My Site host, which isn’t needed.
A while ago, I posted about a problem deploying declarative XML content types and fields.
Error occurred in deployment step ‘Activate Features’: Key cannot be null. Parameter name: Key
I’ve just encountered the same error - but this time with a field being deployed programmatically - i.e., no XML at all. I was deploying a Managed Metadata field (TaxonomyField) and doing this:
TaxonomyField taxField = web.Fields.CreateNewField("TaxonomyFieldType", "My Field") as TaxonomyField; taxField.SspId = termStore.Id; ...snip... taxField.Update(); web.Fields.Add(taxField);
This seemed pretty reasonable - except for the error, of course. In this case it was caused by taxField.Update() - this is not required - the field doesn’t actually exist at this point.
Read more
You may encounter this issue when trying to deploy a Custom Expiration Formula for use as part of records management within SharePoint 2010. The error you may encounter is:
The SPPersistedObject, PolicyConfigService Name=PolicyConfigService, could not be updated because the current user is not a Farm Administrator.
This is a really nasty little error and it may be easy to miss as people have a tendency to use an empty try catch {} in their FeatureActivation so the feature activates but you’re not aware there is a problem. (Well, you become aware of it when for all the activation in the world, the “Use Custom Retention Formula” dropdown never changes from disabled.) It’s particularly annoying because you’re activating a feature at the Site Collection level - why would you need Farm Admin to do it? Well, it’s because it makes changes to the Content Service (or thereabouts) - and this is proven when you go to Central Admin > Security > Configure Information Policy Management > Retention and you’ll notice (after a successful activation) that your custom policy shows up in the “Resources” section.
Read more